Front-End Website Development Courses
Course Duration: 3 Months
Training Period: 2 Hrs Per Day (Monday to Friday)
Training Mode: Online / Offline / Hybrid (One to one Training)
Schedule:
Contact us at info@techaedu.com
Requisites
● Bring your laptop (Windows, Linux or Mac)
● No experience required
Topics covered
-
Introduction to the World Wide Web
-
History of the Web Client-server Architecture
-
Anatomy of a URL
-
HTTP Basics
-
The browser and its Developer Tools
-
Introduction to Front-end Technologies
HTML BASICS
Organizing the Content of a Web Page in HTML
-
Basic Tags and Attributes - Adding Paragraphs, Heading and Links
-
Block-level and Inline Container Tags
-
Including Media Elements - Images, Audio, Video
-
Creating Tables and Lists
-
Adding Forms to Web Pages
-
Form Validation (including usage of HTML5 input elements and attributes)
-
Web Storage - Cookies, Local and Session Storage
-
Web Hosting
CSS BASICS
Understanding the way CSS rules behave
-
Various Ways of Applying Styles
-
CSS Selectors
-
Inheritance of Styles
-
Selector Specificity and the Cascade
CSS Properties
-
The Box Model and related properties
-
Background related properties
-
Text and font related properties
-
Hiding and showing elements
-
Handling horizontal and vertical overflow of content
Layout techniques
-
The display Property
-
Floats
-
Positioning
-
Media Queries and Responsive Web Design
JAVASCRIPT BASICS
Introduction to JavaScript Language Fundamentals
-
Variables and Primitive Data Types
-
Variable Scopes, Scope chain
-
Using Arrays
-
Expressions, Operators and Operator Precedence
-
Control flow - Branching and Looping
Introduction to Functions
-
Function Declaration and Usage
-
Handling Variable Number of Arguments
-
Callbacks - Passing Functions as Arguments
-
Returning Functions
-
Closures
Introduction to Objects
-
Object Declaration using Literal Syntax
-
Accessing Properties and Methods
-
Adding and Deleting Properties
-
The Module Pattern
The “Class” in JavaScript
-
Functions Context (this keyword) and the Constructor Function
-
Introduction to the Object Prototype and Prototype Chain
Basic Introduction to Built-in Classes and Objects in the JavaScript Language
-
call() and apply() as Methods of Functions
-
Array methods
-
Date methods
-
JSON
Introduction to Handling error scenarios
-
Strict Mode Execution
-
Exception Handling using try..catch..finally and throw
The Document Object Model (DOM)
-
Nodes and the DOM Tree
-
Node Relationships and DOM Tree Traversal
-
Methods for DOM Manipulation
Event Handling
-
Various Browser Events
-
Different Ways to Handle Events
-
Event Object Properties and Methods
JQUERY BASICS
Introduction to the Basics of jQuery
-
Why jQuery?
-
DOM Manipulation in jQuery
-
Event Handling in jQuery
-
Ajax requests using jQuery
-
Important Utility Methods
BOOTSTRAP
Bootstrap Fundamentals
-
Introduction to Bootstrap
-
The grid system
-
Typography-related classes
-
Image helper classes
-
Responsive utilities
-
Using Glyph icons and Font awesome
-
Buttons
-
Tables
-
Dropdowns, input groups
-
Alerts
Advanced components
-
Forms
-
Navs and Navbar
-
Panels, collapsible panels and panel groups
-
Modal dialogs
-
Carousel
Theming
-
Introduction to Sass
-
Customizing Bootstrap
ANGULAR
Pre-requisites
-
Introduction to ES6
-
TypeScript Basics
Introduction to Angular
-
The SystemJS Universal Module Loader
-
Directives
-
Routing - Passing data, child routes, and lazy-loading of modules
Dependency injection
-
Injectors and providers
-
Injecting the Http service
-
Communicating with the backend using Http
-
Hierarchy of injectors
Data binding
-
Binding events
-
Binding to properties and attributes
-
2-way data binding
-
Observables and observers
Communication between components
-
Communication patterns
-
Component lifecycle
-
Change detection
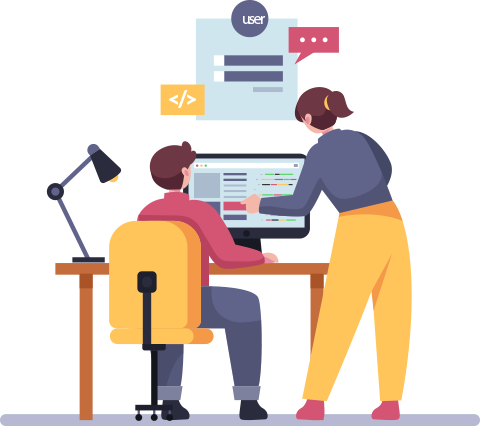